Storing objects is very essential in any programming language and that’s the basic concept behind sets. In this article, I’ll be explaining sets and how it works in JavaScript.
Sets objects lets you store unique values of any type, whether primitive values or object references. Sets are collections of values and those values in the set may only occur once; it is unique in the set’s collection.
In order for you to create a new set in JavaScript, you’ll need to use this syntax:
const keyboard = new Set();

The code above will create an empty set called keyboard.
SET INSTANCE PROPERTIES
- Set.prototype.constructor — The constructor function that created the instance object. For Set instances, the initial value is the Set constructor.
- Set.prototype.size — Returns the number of values in the Set object.
const keyboard = new Set();
keyboard.add("A");
keyboard.add("B");
keyboard.add("C");
keyboard.add("D");
keyboard.add("E");
keyboard.add("F");
keyboard.add("G");
keyboard.add("G");
keyboard.add("G");
console.log(keyboard.size);

SET INSTANCE METHODS
- Set.prototype.add() — Insets a new element with a specified value in a Set object, if there isn’t an element with the same value already in the Set.
const keyboard = new Set();
keyboard.add("A");
keyboard.add("B");
keyboard.add("C");
keyboard.add("D");
keyboard.add("E");
keyboard.add("F");
keyboard.add("G");
keyboard.add("G");
keyboard.add("G");
console.log(keyboard);
2. Set.prototype.clear() — Removes all elements from the Set object
const keyboard = new Set();
keyboard.add("A");
keyboard.add("B");
keyboard.add("C");
keyboard.add("D");
keyboard.add("E");
keyboard.add("F");
keyboard.add("G");
keyboard.add("G");
keyboard.add("G");
console.log(keyboard);
keyboard.clear();
console.log(keyboard);

3. Set.prototype.delete() — Removes the element associated to the value and returns a boolean asserting whether an element was successfully removed or not. Set.prototype.has(value) will return false afterwards.
const keyboard = new Set();
keyboard.add("A");
keyboard.add("B");
keyboard.add("C");
keyboard.add("D");
keyboard.add("E");
keyboard.add("F");
keyboard.add("G");
keyboard.add("G");
keyboard.add("G");
keyboard.delete("A");
console.log(keyboard);

4. Set.prototype.entries() — Returns a new set iterator object that contains an array of [value, value] for each element in the Set object, in insertion order. For Set objects there is not key like in Map objects. However, to keep the API similar to the Map object, each entry has the same value for its key and value here, so that an array [value, value] is returned.
const keyboard = new Set();
keyboard.add("A");
keyboard.add("B");
keyboard.add("C");
keyboard.add("D");
keyboard.add("E");
keyboard.add("F");
keyboard.add("G");
keyboard.add("G");
keyboard.add("G");
const iterator = keyboard.entries();
for (const entry of iterator) {
console.log(entry);
}

5. Set.prototype.forEach() — Calls callbackFn once for each value present in the Set object, in insertion order. If a thisArg parameter is provided, it will be used as the this value for each invocation of callbackFn.
const keyboard = new Set();
keyboard.add("A");
keyboard.add("B");
keyboard.add("C");
keyboard.add("D");
keyboard.add("E");
keyboard.add("F");
keyboard.add("G");
keyboard.add("G");
keyboard.add("G");
function eachKeys(value1, value2, set) {
console.log(`s[${value1}] = ${value2}`);
}
new Set(["I", "J", undefined]).forEach(eachKeys);

6. Set.prototype.has() — Returns a boolean asserting whether an element is present with the given value in the Set object or not.
const keyboard = new Set();
keyboard.add("A");
keyboard.add("B");
keyboard.add("C");
keyboard.add("D");
keyboard.add("E");
keyboard.add("F");
keyboard.add("G");
keyboard.add("G");
keyboard.add("G");
console.log(keyboard.has("D"));
console.log(keyboard.has("I"));

7. Set.prototype.keys() — An alias for Set.prototype.values()
8. Set.prototype.values() — Returns a new iterator object that yields the values for each element in the Set object in insertion order.
const keyboard = new Set();
keyboard.add("A");
keyboard.add("B");
keyboard.add("C");
keyboard.add("D");
keyboard.add("E");
keyboard.add("F");
keyboard.add("G");
keyboard.add("G");
keyboard.add("G");
const iterator = keyboard.values();
console.log(iterator.next().value);
console.log(iterator.next().value);
console.log(iterator.next().value);
console.log(iterator.next().value);
console.log(iterator.next().value);

9. Set.prototype[@@iterator]() — Returns a new iterator object that yields the values for each element in the Set object in insertion order.
MORE EXAMPLES
To iterate sets
const mySet1 = new Set();
mySet1.add(1);
mySet1.add(5);
mySet1.add(5);
mySet1.add("some text");
const o = { a: 1, b: 2 };
mySet1.add(o);
mySet1.add({ a: 1, b: 2 });
for (const iterate of mySet1.values()) {
console.log(iterate);
}
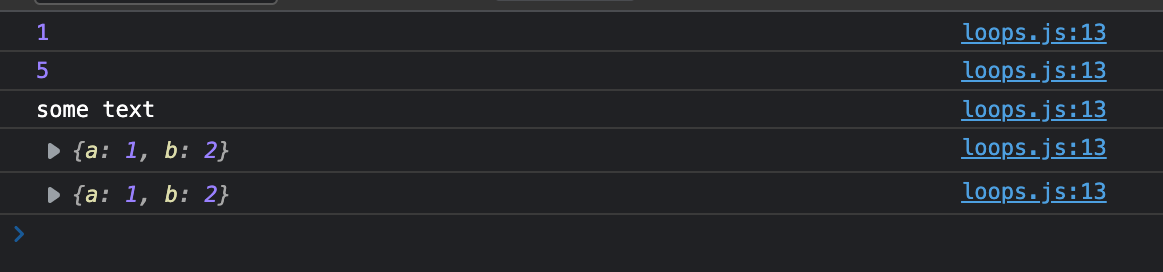
To convert set object to an array object
const mySet1 = new Set();
mySet1.add(1);
mySet1.add(5);
mySet1.add(5);
mySet1.add("some text");
const o = { a: 1, b: 2 };
mySet1.add(o);
mySet1.add({ a: 1, b: 2 });
const myArr = Array.from(mySet1);
console.log(myArr);

To convert from set to array
const mySet1 = new Set();
mySet1.add(1);
mySet1.add(5);
mySet1.add(5);
mySet1.add("some text");
const o = { a: 1, b: 2 };
mySet1.add(o);
mySet1.add({ a: 1, b: 2 });
const mySet2 = new Set([1, 2, 3, 4]);
console.log(mySet2.size);
console.log([...mySet2]); // using the spread syntax ...

To simulate intersection
const mySet1 = new Set();
mySet1.add(1);
mySet1.add(5);
mySet1.add(5);
mySet1.add("some text");
const o = { a: 1, b: 2 };
mySet1.add(o);
mySet1.add({ a: 1, b: 2 });
const mySet2 = new Set([1, 2, 3, 4]);
const intersection = new Set([...mySet1].filter((x) => mySet2.has(x)));
console.log(intersection);

To simulate difference
const mySet1 = new Set();
mySet1.add(1);
mySet1.add(5);
mySet1.add(5);
mySet1.add("some text");
const o = { a: 1, b: 2 };
mySet1.add(o);
mySet1.add({ a: 1, b: 2 });
const mySet2 = new Set([1, 2, 3, 4]);
const intersection = new Set([...mySet1].filter((x) => !mySet2.has(x)));
console.log(intersection);

To iterate set entries
const mySet1 = new Set();
mySet1.add(1);
mySet1.add(5);
mySet1.add(5);
mySet1.add("some text");
const o = { a: 1, b: 2 };
mySet1.add(o);
mySet1.add({ a: 1, b: 2 });
const mySet2 = new Set([1, 2, 3, 4]);
const intersection = new Set([...mySet1].filter((x) => !mySet2.has(x)));
mySet2.forEach((value) => {
console.log(value);
});

Implementing basic operations
function isAnimal(a, b) {
for (const fur of b) {
if (!a.has(fur)) {
return false;
}
}
return true;
}
function marriage(a, b) {
const _union = new Set(a);
for (const kiss of b) {
_union.add(kiss);
}
return _union;
}
function intersection(a, b) {
const _intersection = new Set();
for (const elem of b) {
if (a.has(elem)) {
_intersection.add(elem);
}
}
return _intersection;
}
function symmetricDifference(a, b) {
const _difference = new Set(a);
for (const elem of b) {
if (_difference.has(elem)) {
_difference.delete(elem);
}
else {
_difference.add(elem);
}
}
return _difference;
}
function difference(a, b) {
const _difference = new Set(a);
for (const elem of b) {
_difference.delete(elem);
}
return _difference;
}
const a = new Set([1, 2, 3, 4]);
const b = new Set([2, 3]);
const c = new Set([3, 4, 5, 6]);
console.log(isAnimal(a, b));
console.log(marriage(a, b));
console.log(intersection(a, b));
console.log(symmetricDifference(a, b));
console.log(difference(a, b));

Set relations to arrays
const myArray = ["A", "B", "C", "D", "E", "F", "G"];
const mySet = new Set(myArray);
console.log(mySet);
console.log(mySet.has("A"));
console.log([...mySet]);

Removing duplicate elements from an array
const keyboard = [1, 2, 4, 4, 6, 7, 4, 6, 8, 6, 2, 7, 10, 23, 14, 18];
console.log([...new Set(keyboard)]);

Relation to strings
const country = "Nigeria";
const mySet = new Set(country);
console.log(mySet);
console.log(mySet.size);
console.log(new Set("NIGeria"));
console.log(new Set("nigeria"))

I hope by now you’ve understand the concepts behind set in JavaScript. This resources was made possible by the mozilla documentation which I’ve personally been using so far. If you’re looking to learn about JavaScript as a beginner, I highly recommend you check it out.
I hope this article has been so helpful to you. Don’t forget to give me a clap 👏 share this article & follow me on my social media handles: twitter , facebook and youtube.